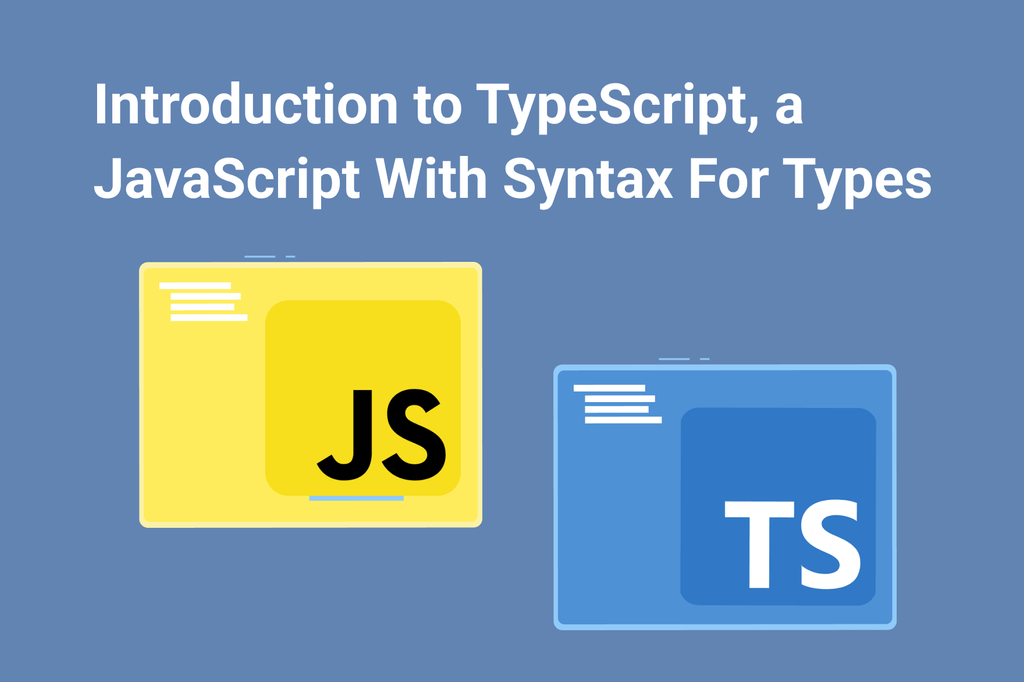
FAQ
What is the difference between JavaScript and TypeScript?
TypeScript is an open-source object-oriented programming language developed by Microsoft Corporation, whereas JavaScript is the programming language for the web. TypeScript is designed to build large-scale web apps, whereas JavaScript is a server-side programming language that helps to develop interactive web pages.
What are the two types of TypeScript?
The TypeScript type system has two special types, null and undefined , that have the values null and undefined respectively. We mentioned these briefly in the Basic Types section. By default, the type checker considers null and undefined assignable to any type. Effectively, there is no value of any type that is not implicitly assignable to null or undefined.
What exactly is TypeScript?
TypeScript is a programming language that adds static typing to JavaScript. Static typing basically means that types are added to the code, allowing developers to define and use types. The phrase "Syntactic Superset" simply means that TypeScript shares the same base syntax as JavaScript, but adds something extra on top of that.
Table of contents:
Want to estimate your app idea?