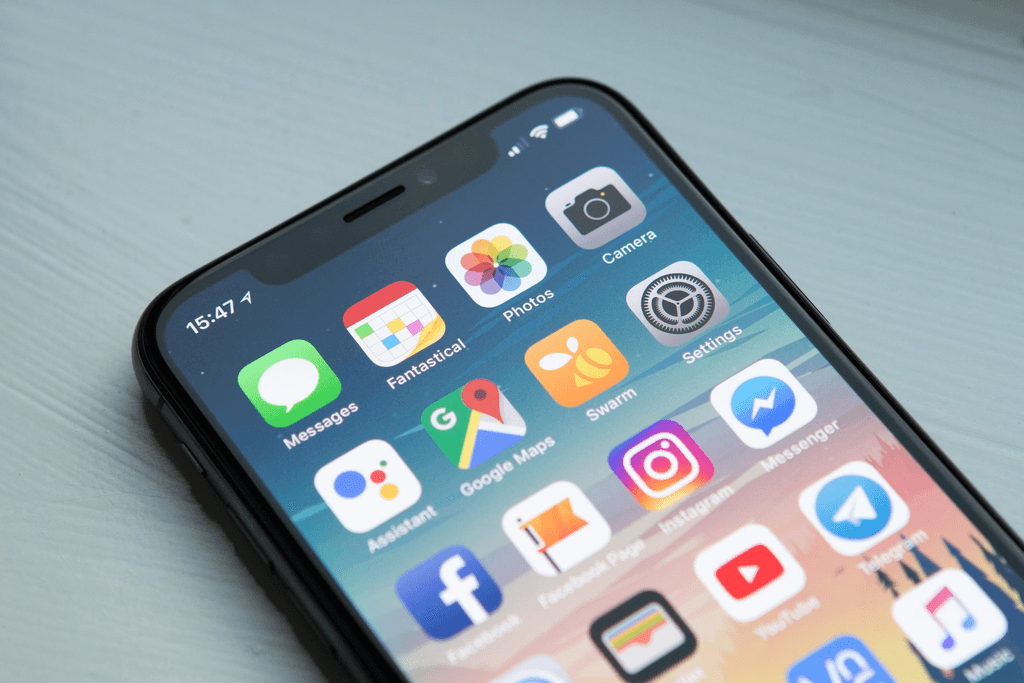
FAQ
How does the auto layout system work in iOS?
Auto Layout is a system in iOS that you can use to create user interfaces for your app. When you design an interface, you typically define its layout by creating constraints between the various elements. Auto Layout then calculates the size and location of each element based on these constraints. This allows your app to dynamically respond to both internal and external changes.
How do I use auto layout effectively in iOS?
In some cases, setting constraints programmatically might be your only option. If you want to change the layout of your views at runtime, for example, you might not be able to use Interface Builder. There are most important features of Auto Layout: orientation-based layout, size classes, constraint priorities, proportional constraints, and content hugging and compression.
Is iOS programming challenging to learn?
Swift is a relatively straightforward language to learn, and with helpful feedback, you can reach the point where you can begin doing useful things with it. However, although knowing Swift or Objective-C makes you somewhat of a Developer, there's still much to learn before you can call yourself one.
Table of contents:
Want to estimate your app idea?