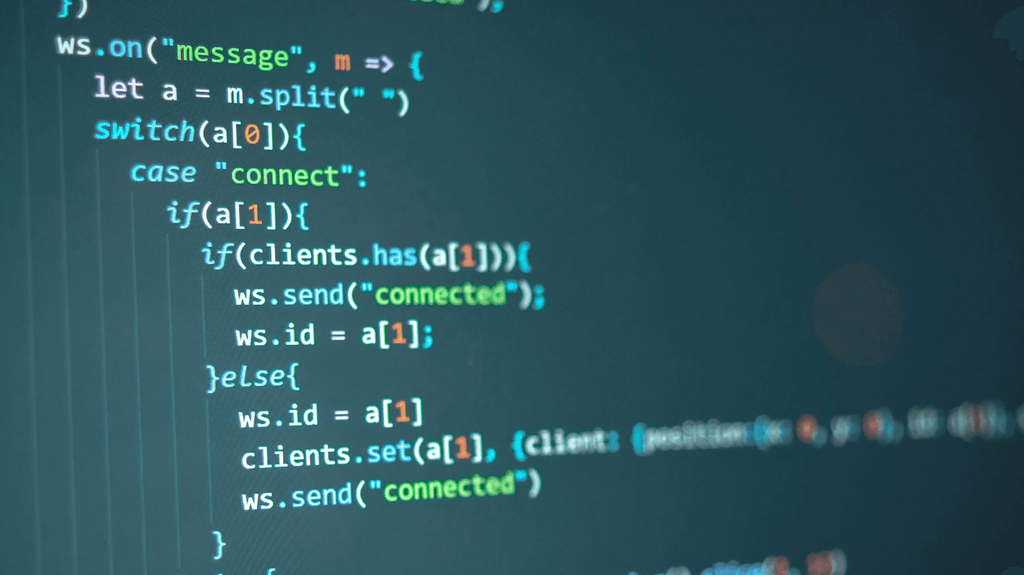
FAQ
Are WebSockets a real-time technology?
The primary function of TCP is to provide a bi-directional communication channel for data transmission. This allows a browser and a web server to interact, providing them with a two-way persistent connection, within which they can send messages back and forth while the connection is open.
When would it be appropriate to use WebSockets?
WebSockets should be used when two-way communication is needed between two networked systems, for example when building a chat application. WebSockets are also useful for data visualization dashboards or maps that need to reflect real-time data values.
What language is best for developing WebSockets?
A WebSocket server can be written in any server-side language that supports the Berkeley socket API, such as C(++), Python, PHP or JavaScript.
Table of contents:
Want to estimate your app idea?